Survey Embedding
Embed zeffi surveys into your webpage as a part of the page via iframe, or as a popup survey. Communicate with your surveys using our easy client API.
Embedding via iframe
You can embed the survey via iframe using the following sample code.
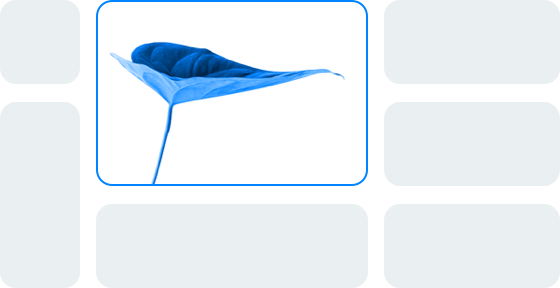
Popup via JavaScript
To create a popup survey, put the following script inside your page header tag.
Popup functions
Opens the survey popup. In case of multiple keys, the first survey is opened.
Opens a specific survey popup, identified by the KEY. There can only be one survey opened at a time, this closes any other survey if currently open.
Closes the currently open popup.
Toggles the open / close state of the popup. In case of multiple keys, the first survey is opened.
Toggles the open / close state of the popup. When opening, the specific survey is opened identified by the KEY.
Shows the clickable bubble. In case of multiple keys, clicking the bubble opens the first survey.
Shows the clickable bubble. Clicking the bubble opens the specific survey identified by the KEY.
Hides the clickable bubble if visible.
Note: Without bubble the user cannot open the popup, and it needs to be opened via code.
Abstract models
// this will restart the survey with a new answerer instance
restart?: boolean;
// language code to start the survey with (eg. 'fi')
language?: string;
// start in accessibility mode
accessibilityMode?: boolean;
// zeffi contact identifier
zefId?: string
// background information to attach to the answerer session
respondentFields?: Record<string, string>;
}
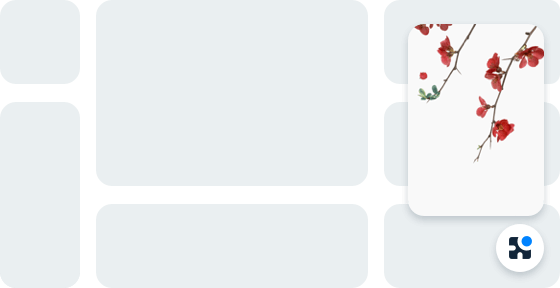
Survey communication
Make your embed or popup talk with your website by listening to events.
The wildcard (*) is allowed, but strongly discouraged.
Read more here about the proper usage.
function onMessageReceived(message) {
if (message.origin === 'https://survey.zef.fi') {
// you have received a message from the survey
// use message.data.type to get the message type
// use message.data.data to get the message payload
}
}
Example code to listen for an event
Abstract models
type: SurveyEventType;
data: SurveyEventData;
}
The data property on all events sent from the survey will have the SurveyEvent structure.
team: string;
survey: string;
linkKey: string;
answererId: string;
timestamp: number;
}
Base data sent with every event.
Start = 'start',
Answer = 'answer',
Complete = 'complete',
Restart = 'restart',
Outcome = 'outcome'
}
The event type, set on the SurveyEvent.
Possible events
start
Will be emitted when a survey is started.
answer
question: QuestionData;
answer: string;
progress: number;
answerType: string;
}
Will be emitted when an answer is given.
complete
progress: number;
}
Will be emitted when a survey is completed.
outcome
outcomes: SurveyOutcomeData[];
}
interface SurveyOutcomeData {
score: number;
outcome: OutcomeData;
}
Will be emitted when a survey outcome changes. This will be fired throughout the answering of the survey.
restart
Will be emitted when a survey is restarted.